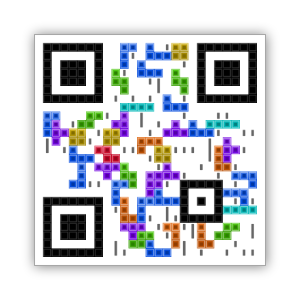
The Ultimate QR Editor
Iterate over all encodings
type
(String)value
(String or Object)options
(Object)Returns: { total, get }
total
: number of permutationsget(i)
: get i-th permutation for 0 <= i < totallet permutations = permute('url','https://qrs.art',{
protocolCaps:true,
domainCaps:false,
pathCaps:false
})
permutations.total // ~> 32
permutations.get(0) // ~> https://qrs.art/
permutations.get(permutations.total-1) // ~> HTTPS://qrs.art/
for(let str of permutations){
console.log(str)
}
"https://qrs.art/","Https://qrs.art/",
"hTtps://qrs.art/","HTtps://qrs.art/",
"htTps://qrs.art/","HtTps://qrs.art/",
"hTTps://qrs.art/","HTTps://qrs.art/",
"httPs://qrs.art/","HttPs://qrs.art/",
"hTtPs://qrs.art/","HTtPs://qrs.art/",
"htTPs://qrs.art/","HtTPs://qrs.art/",
"hTTPs://qrs.art/","HTTPs://qrs.art/",
"httpS://qrs.art/","HttpS://qrs.art/",
"hTtpS://qrs.art/","HTtpS://qrs.art/",
"htTpS://qrs.art/","HtTpS://qrs.art/",
"hTTpS://qrs.art/","HTTpS://qrs.art/",
"httPS://qrs.art/","HttPS://qrs.art/",
"hTtPS://qrs.art/","HTtPS://qrs.art/",
"htTPS://qrs.art/","HtTPS://qrs.art/",
"hTTPS://qrs.art/","HTTPS://qrs.art/"
URLs must start with (https/http). Permuting upper/lower case of protocol and domain are generally safe. If your server is case-insensitive, you can also enable pathCaps.
permute('url','https://qrs.art',{
protocolCaps:true,
domainCaps:false,
pathCaps:false
})
WIFI:T:WPA;S:Guest Wifi;P:1234;;
permute('wifi',{name:'Guest Wifi',password:'1234'},{
keyCaps:true,
})