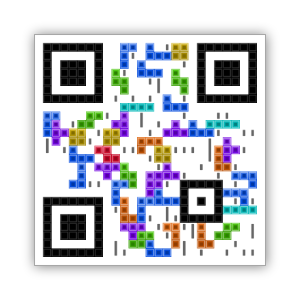
The Ultimate QR Editor
Utility class for 2D patterns
new Grid(w,h)
w
: int widthh
: int heightx
: col indexy
: row indexv
: truthy or falsy valuex
: col indexy
: row indexx
: col indexy
: row indexReturns: [x,y]
iterator with value matching v
v == null
, returns all used
coordinatesv == true
, returns all on
coordinatesv == false
, returns all off
coordinatesimport { Grid } from 'qrsart'
let grid = new Grid(10,10)
grid.set(4,4,1)
grid.set(4,3,0)
grid.set(3,3,true)
grid.set(3,4,false)
for(let [x,y] of grid.tiles()){
ctx.fillStyle = grid.get(x,y) ? 'red' : 'blue'
ctx.fillRect(x,y,1,1)
}
Returns: Grid
Combine all used coordinates. If any are true
, cell becomes true
/
Returns: Grid
Removes used coordinates in any of grids from og.
Returns: Grid
Only include common cells.